Tinker Board 2 series:
C:
C is a general-purpose, imperative computer programming language, supporting structured programming, lexical variable scope and recursion, while a static type system prevents many unintended operations.
Ps: The GPIO WiringPi for C library has been installed in Tinker Board 2 series by default. Step 1 and 2 can be ignored.
- Navigate to folder
cd /usr/local/share/gpio_lib_c_rk3399
- Install C GPIO library for Tinker Board 2 series
sudo ./build
- Check install success or not
gpio readall
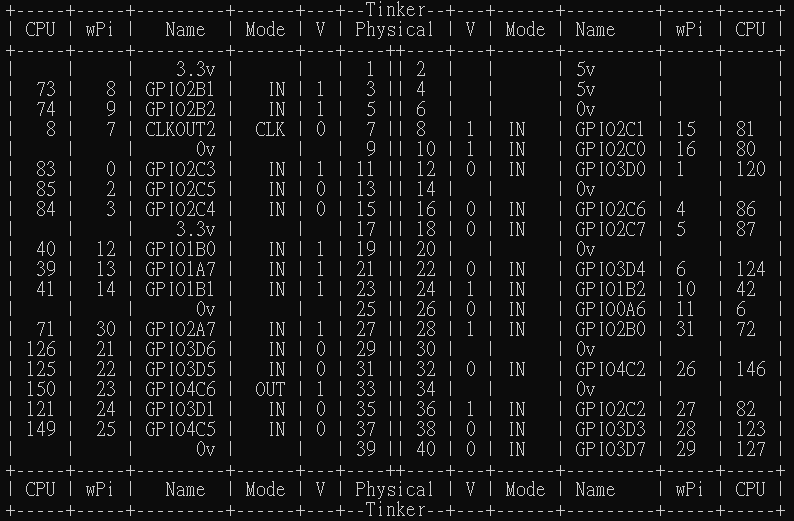
- Reference codes
There’re few sample codes under this folder
/usr/local/share/gpio_lib_c_rk3399/examples
To make a simple script create a file with ‘nano blink.c’ and input the following code.
Sample LED blink: Please reference the pin map results about gpio readall.
#include <stdio.h>
#include <wiringPi.h>
// LED Pin - wiringPi pin 30 is GPIO2A7 71.
#define LED 30
int main (void)
{
printf ("Tinker board 2 blink\n") ;
wiringPiSetup () ;
pinMode (LED, OUTPUT) ;
for (;;)
{
digitalWrite (LED, HIGH) ; // On
delay (500) ; // mS
digitalWrite (LED, LOW) ; // Off
delay (500) ;
}
return 0 ;
}
To run the script run the command:
sudo gcc -o blink blink.c -lwiringPi
To run the newly compiled led run the command ‘sudo ./blink
’
.sh file for Tinker_2S_Test_input_gpio: https://github.com/TinkerBoard/TinkerBoard/files/8109019/Tinker_2S_Test_input_gpio.zip
Python:
Python is a programming language that lets you work quickly and integrate systems more effectively.
Ps: The GPIO WiringPi for Python library has been installed in Tinker Board 2 series by default. Step 1 and 2 can be ignored.
Please refer to the pin mapping table above
Navigate to folder
cd /usr/local/share/gpio_lib_python_rk3399
- Install Python GPIO library for Tinker Board 2 series for python and python3
sudo python setup.py install
sudo python3 setup.py install
- Reference codes
There’re few sample codes under this folder
cd /usr/local/share/gpio_lib_python_rk3399/test
To check the sample code by ‘nano forloop.py
’ and you can see the following codes.
Sample forloop functions: set the all gpio value to high and then set all gpio value to low
import ASUS.GPIO as GPIO
import unittest
import time
# to use ASUS tinker board pin numbers
GPIO.setmode(GPIO.BOARD)
# set up GPIO output channel, we set GPIO4 (Pin 7) to OUTPUT
n = 40
for counter in range (1, n+1):
if counter == 1 or \
counter == 2 or \
counter == 4 or \
counter == 6 or \
counter == 9 or \
counter == 14 or \
counter == 17 or \
counter == 20 or \
counter == 25 or \
counter == 30 or \
counter == 34 or \
counter == 39 :
continue
GPIO.setup(counter, GPIO.OUT)
GPIO.output(counter,GPIO.HIGH)
time.sleep(1)
for counter in range (1, n+1):
if counter == 1 or \
counter == 2 or \
counter == 4 or \
counter == 6 or \
counter == 9 or \
counter == 14 or \
counter == 17 or \
counter == 20 or \
counter == 25 or \
counter == 30 or \
counter == 34 or \
counter == 39 :
continue
GPIO.output(counter,GPIO.LOW)
time.sleep(1)
To run the python sample code by the following commands:
sudo python forloop.py
Ps: Please reference the pin value by ‘gpio readall’.